Part 2: Write Your Custom Code to Authenticate
With the Salesforce connected app created and configured we are ready to proceed forward with developing our custom app code needed to authenticate from an external application. And, as imagined, you can view this as the right hand of the handshake.
This can be done from various technologies and languages. For this series we’ll be using an open source .NET CMS & application development framework (DNN) to illustrate the needed steps to authenticate. Accordingly, the code written in our app will be written in C# and ASP.NET.
Like Simpplr, DNN is a very extensible platform that allows developers to create custom extensions. The most popular extension type in DNN is the custom module, which is very similar to Simpplr’s tile feature. We will leverage a custom module in DNN for this exercise and I will omit some DNN specific details around development as they are not directly relevant.
As this guide is instructional in nature and in hopes of simplicity, I’ve simply created buttons and labels for each step of the authentication process. We will click a button, make some magic happen, and update a label accordingly. Doing it this way creates a linear flow with steps that can be followed and replicated.
Disclaimer
There are some parts of this code that should be combined to run more efficiently and can be refactored to be more succinct and with less methods present. You should customize and refactor your code as needed. The below code is set up to help convey instructional concepts.
All that said, here’s what we’re about to do:
- Get the Authorization Code
- Use the Authorization Code to get an Access Token
- Use the Access Token to access the Simpplr API
Note:
Salesforce has really good documentation around this process which can be found on this page OAuth 2.0 Web Server Flow for Web App Integration.
So let’s get started…
Getting the Authorization Code
The first step in the authentication process is to get an Authorization Code from Salesforce. To do this we need to hit a specific URL endpoint passing in a few parameters that are unique to our connected application. Those unique parameters are the client_id, redirect_uri, and response_type.
An example of the full URL:
Example URL
In our custom DNN module, the view has a button that, when clicked, will access the aforementioned unique endpoint. The url accessed in this click event has been concatenated to hopefully make it easier to understand and update as shown below.

When the button is clicked and this code is executed the browser is redirected to the specified URL, which will initially prompt the user to allow access to the Salesforce environment on the first time requesting the authorization code. The screen that is shown will look similar to the below:
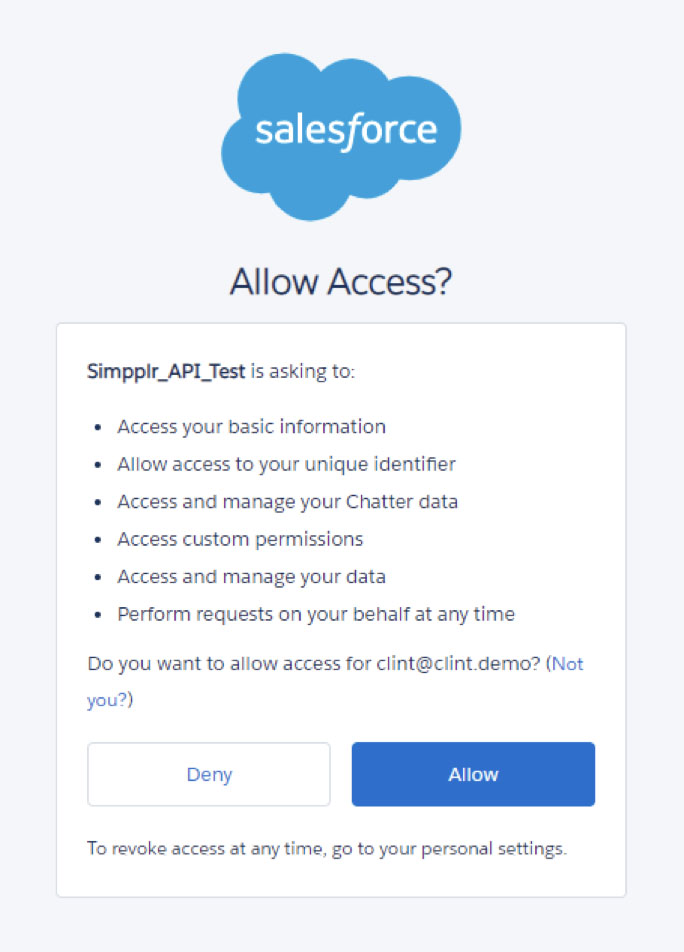
After logging in, the browser redirects the user to the url specified in the Redirect URI parameter along with appending the authorization code as a parameter as shown below...

Now that we have the authorization code we are ready and able to get the access token, which is what is needed to be able to interact with the Simpplr API endpoints.
Getting the Access Token
To get the access token we create an HTTPClient so that we can make an HTTP request. In this request we create a Dictionary of FormURLEncoded content that will send our unique information in the request. Notice the client ID & client secret (that we got when we set up our connected app) included in this request along with the grant_type and authorization code that we just received being included.
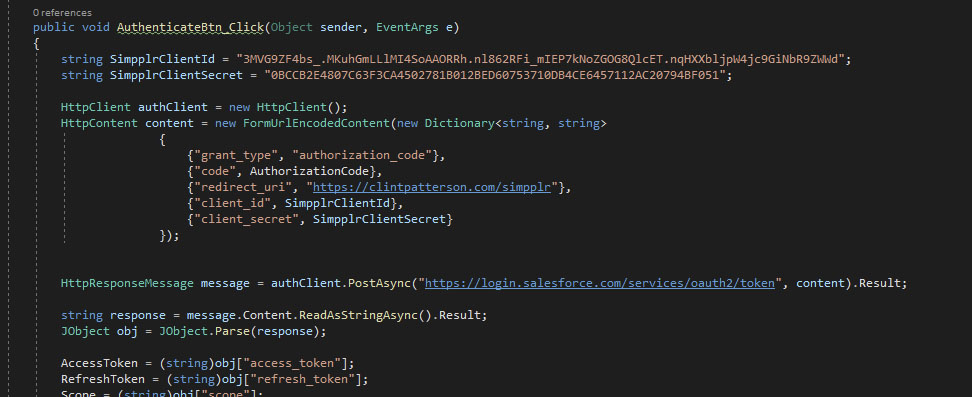
With our request ready, we make an asynchronous request and we parse the response as JSON so that we can work with it. In the example code, I’ve updated labels with all of the info that the request returned. From the response we received an access token, but we also got a refresh token and more informative information. We will use the access token to get info from the Simpplr API endpoint.
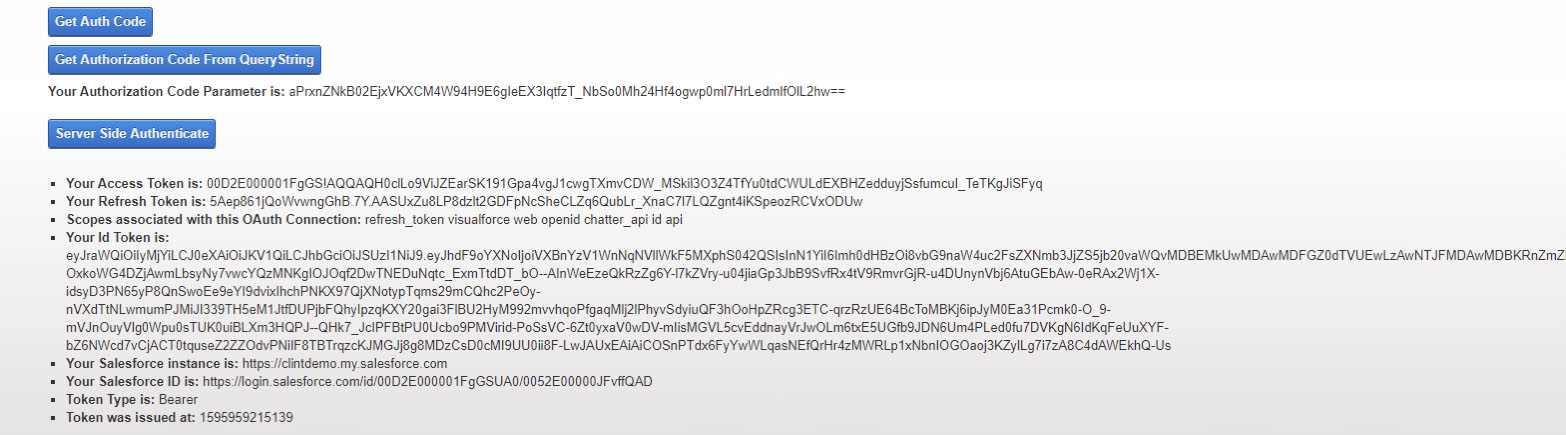
Video Walkthrough
Congrats on Getting Authenticated!
Don’t look now, but you’ve made a lot of progress. Jumping through the authentication hoops are sometimes the most “interesting” parts of development. Hopefully this walkthrough has demystified some of the authentication steps for you.
Next Steps
Now that we’ve got the access token, we are officially authenticated to our connected app and we are ready to move forward with our APIs.